Here’s a comprehensive list of 50 SwiftUI commands:
1. Text
Displays static or dynamic text.
Example:
Displays the text “Hello, World!” on the screen.
2. Button
Creates a tappable button.
Example:

Creates a button labeled “Tap me” that prints a message when tapped.
3. Image
Displays an image from assets or system.
Example:

Displays a filled star image using SF Symbols.
4. VStack
Vertically stacks its child views.
Example:
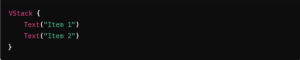
Arranges “Item 1” and “Item 2” vertically.
5. HStack
Horizontally stacks its child views
Example:
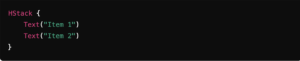
Arranges “Item 1” and “Item 2” horizontally.
6. ZStack
Overlays views on top of each other.
Example:
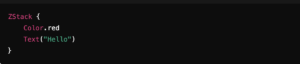
Places “Hello” text on top of a red background.
7. Spacer
Adds flexible spacing between views.
Example:
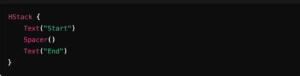
Pushes “Start” and “End” texts to opposite sides.
8. List
Displays a scrollable list of items.
Example:
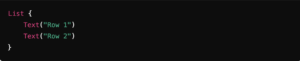
Creates a vertical scrolling list with two rows.
9. ForEach
Dynamically generates views from a collection.
Example:

Creates 5 text items dynamically.
10. NavigationView
Wraps views in a navigation container.
Example:

Displays the content inside a navigation context.
11. NavigationLink
Creates a navigable link to another view.
Example:

Navigates to the “Detail View” when tapped.
12. Form
Creates a form with input fields and other controls.
Example:

Creates a form with a label and text field.
13. TextField
Accepts user input as text.
Example:

Displays an editable text field with a placeholder.
14. SecureField
Creates a password-protected text input.
Example:

Masks the entered text for privacy.
15. Toggle
Creates a switch to enable or disable options.
Example:

Creates a switch to toggle an option on or off.
16. Slider
Creates a range-based slider for value selection.
Example:
Allows users to select a value between 0 and 1.
17. Picker
Presents a selection interface.
Example:
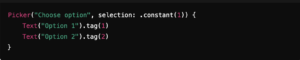
Creates a picker with two options.
18. Step
Provides an incremental counter control.
Example:

Creates a stepper to increment or decrement values.
19. DatePicker
Provides a control for picking a date and/or time.
Example:

Allows users to pick a date.
20. ProgressView
Displays a progress indicator.
Example:

Shows a progress bar indicating 70% completion.
21. Sheet
Presents a modal sheet from the bottom of the screen.
Example:
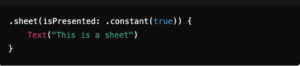
Displays a modal view as a sheet.
22. Alert
Displays an alert with a message and buttons.
Example:

Shows an alert with a warning message.
23. ActionSheet
Displays a set of options in a pop-up from the bottom.
Example:

Presents a set of options for user action.
24. ClipShape
Clips a view into a specific shape.
Example: